暂停线程意味着此线程还可以恢复运行。在Java多线程中,可以使用suspend()方法暂停线程,使用resume()方法恢复线程。
suspend与resume方法的使用
代码清单1 suspend与resume方法的使用
从控制台打印的时间上看,线程的确被暂停了,而且还可以恢复成运行的状态。
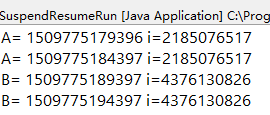
图1 暂停与恢复线程
suspend与resume方法的缺点–独占
1) 在使用suspend与resume方法时,如果使用不当,极易造成公共的同步对象的独占,使得其他线程无法访问公共同步对象。
代码清单2 suspend与resume独占
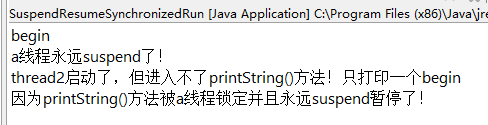
图2 独占并锁死了printString方法
2) 还有另外一种独占锁的情况也要格外注意,稍有不慎,就会掉进“坑”里。
代码清单3 另外一种独占锁的情况
上面输出如下:
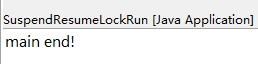
图3 控制台打印main end信息
将代码清单3中SuspendResumeLockThread类中//System.out.println(i);
注释符//
去掉。
再次运行程序,控制台不打印main end,运行结果如下。
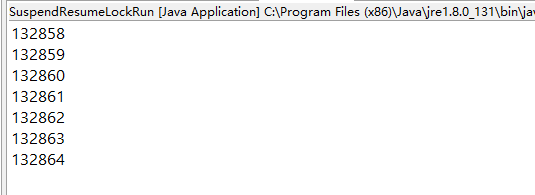
图4 不打印main end信息
出现这样情况的原因是,当程序运行到println()方法内部停止时,同步锁未被释放。方法println()源代码如图5所示。
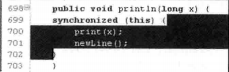
图5 锁不释放
这导致当前PrintStream对象的println方法一直呈”暂停”状态,并且“锁未释放“,而main()方法中的代码System.out.println("main end!");
迟迟不能执行打印。
虽然suspend()方法是国期作废的方法,但还是有必要研究它过期作废的原因,这是很有意义的。
suspend与resume方法的缺点—-不同步
在使用suspend与resume方法时也容易出现线程的暂停而导致数据不同步的情况。
代码清单4 数据不同步
程序运行的结果出现值不同步的情况,所以在程序中使用suspend()方法要格外注意。
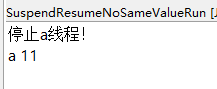
图6 运行结果